Word to PDF Conversion Automation
Serving as a virtual printer, Universal Document Converter will be able to save your documents as PDF, TIFF, JPEG, or PNG files.
Software developers can use the COM-interface and Microsoft Word as COM-server for converting Word to PDF.
Word document conversion source code examples:
Visual Basic.NET
VB.NET Word to PDF conversion code example:
'----------------------------------------------------------------- ' 1) Microsoft Word version 97 or higher should be installed ' and activated on your PC. ' ' 2) Universal Document Converter 5.2 or higher should be installed as well ' ' 3) Open your project in Microsoft Visual Basic.NET ' ' 4) In Visual Basic main menu press Project->Add Reference... ' ' 5) In Add Reference window go to COM tab and double click ' into Universal Document Converter Type Library. '----------------------------------------------------------------- Private Sub PrintWordToPDF(ByVal strFilePath As String) Dim objUDC As UDC.IUDC Dim itfPrinter As UDC.IUDCPrinter Dim itfProfile As UDC.IProfile Dim WordApp As Object Dim WordDoc As Object objUDC = New UDC.APIWrapper itfPrinter = objUDC.Printers("Universal Document Converter") itfProfile = itfPrinter.Profile ' Use Universal Document Converter API to change settings of converterd document itfProfile.PageSetup.ResolutionX = 600 itfProfile.PageSetup.ResolutionY = 600 itfProfile.FileFormat.ActualFormat = UDC.FormatID.FMT_PDF itfProfile.FileFormat.PDF.Multipage = UDC.MultipageModeID.MM_MULTI itfProfile.OutputLocation.Mode = UDC.LocationModeID.LM_PREDEFINED itfProfile.OutputLocation.FolderPath = "C:\Out" itfProfile.OutputLocation.FileName = "&[DocName(0)].&[ImageType]" itfProfile.OutputLocation.OverwriteExistingFile = False ' Run Microsoft Word as COM-server WordApp = CreateObject("Word.Application") ' Open document from file WordDoc = WordApp.Documents.Open(strFilePath, , 1) ' Print all pages of the document WordApp.ActivePrinter = "Universal Document Converter" Call WordApp.PrintOut(False) ' Close the document Call WordDoc.Close() WordDoc = Nothing ' Close Microsoft Word Call WordApp.Quit() WordApp = Nothing End Sub
Visual Basic 6
VB Word to PDF conversion code example:
'----------------------------------------------------------------- ' 1) Microsoft Word 97 or higher should be installed and activated on your PC ' ' 2) Universal Document Converter 5.2 or higher should be installed as well ' ' 3) Open your project in Microsoft Visual Basic 6.0 ' ' 4) In Visual Basic main menu press Project->References ' ' 5) In the list of references check ' Universal Document Converter Type Library. '----------------------------------------------------------------- Private Sub PrintWordToPDF(strFilePath As String) Dim objUDC As IUDC Dim itfPrinter As IUDCPrinter Dim itfProfile As IProfile Dim WordApp As Object Dim WordDoc As Object Set objUDC = New UDC.APIWrapper Set itfPrinter = objUDC.Printers("Universal Document Converter") Set itfProfile = itfPrinter.Profile ' Use Universal Document Converter API to change settings of converterd document itfProfile.PageSetup.ResolutionX = 600 itfProfile.PageSetup.ResolutionY = 600 itfProfile.FileFormat.ActualFormat = FMT_PDF itfProfile.FileFormat.PDF.Multipage = MM_MULTI itfProfile.OutputLocation.Mode = LM_PREDEFINED itfProfile.OutputLocation.FolderPath = "C:\Out" itfProfile.OutputLocation.FileName = "&[DocName(0)].&[ImageType]" itfProfile.OutputLocation.OverwriteExistingFile = False ' Run Microsoft Word as COM-server On Error Resume Next Set WordApp = CreateObject("Word.Application") ' Open document from file Err = 0 ' Clear GetLastError() value Set WordDoc = WordApp.Documents.Open(strFilePath, , 1) If Err = 0 Then ' Print all pages of the document WordApp.ActivePrinter = "Universal Document Converter" Call WordApp.PrintOut(False) ' Close the document Call WordDoc.Close Set WordDoc = Nothing End If ' Close Microsoft Word Call WordApp.Quit Set WordApp = Nothing End Sub
Visual C++
C++ Word to PDF conversion code example:
////////////////////////////////////////////////////////////////// // This example was designed to be used in Microsoft Visual C++ // from Microsoft Visual Studio 2003 or higher. // // 1. Microsoft Word 97 or higher should be installed // and activated on your PC. // // 2. Universal Document Converter 5.2 or higher should be // installed as well. // // 3. You must initialize the COM before you call any COM method. // Please insert ::CoInitialize(0); in your application // initialization and ::CoUninitialize(); before closing it. // // 4. Import Office libraries for 32-bit version of Windows. // For 64-bit version please change C:\\Program Files\\ to // C:\\Program Files (x86)\\ in all pathes. #pragma message("Import MSO.DLL") // MS Office 2000 -> // C:\\Program Files\\Common Files\\Microsoft Shared\\OFFICE10\\MSO.DLL // // MS Office 2003 -> // C:\\Program Files\\Common Files\\Microsoft Shared\\OFFICE11\\MSO.DLL // // MS Office 2007 -> // C:\\Program Files\\Common Files\\Microsoft Shared\\OFFICE12\\MSO.DLL #import "C:\\Program Files\\Common Files\\Microsoft Shared\\OFFICE12\\MSO.DLL" \ rename_namespace("MSO"), auto_rename #pragma message("Import VBE6EXT.OLB") #import "C:\\Program Files\\Common Files\\Microsoft Shared\\VBA\\VBA6\\VBE6EXT.OLB" \ rename_namespace("VBE6EXT") #pragma message("Import MS Word API") // MS Office 2000 -> "C:\\Program Files\\Microsoft Office\\OFFICE\\MSWORD9.OLB" // MS Office 2003 -> "C:\\Program Files\\Microsoft Office\\OFFICE11\\MSWORD.OLB" // MS Office 2007 -> "C:\\Program Files\\Microsoft Office\\OFFICE12\\MSWORD.OLB" #import "C:\\Program Files\\Microsoft Office\\OFFICE12\\MSWORD.OLB" \ rename_namespace("MSWORD"), auto_rename // 5. Import Universal Document Converter software API: #import "progid:udc.apiwrapper" rename_namespace("UDC") ////////////////////////////////////////////////////////////////// static COleVariant vTrue( (short)TRUE ), vFalse( (short)FALSE ); static COleVariant vOpt( (long)DISP_E_PARAMNOTFOUND, VT_ERROR ); void PrintWordToPDF(CString szFilePath) { UDC::IUDCPtr pUDC(__uuidof(UDC::APIWrapper)); UDC::IUDCPrinterPtr itfPrinter = pUDC->Printers["Universal Document Converter"]; UDC::IProfilePtr itfProfile = itfPrinter->Profile; // Use Universal Document Converter API to change settings of converterd document itfProfile->PageSetup->ResolutionX = 600; itfProfile->PageSetup->ResolutionY = 600; itfProfile->FileFormat->ActualFormat = UDC::FMT_PDF; itfProfile->FileFormat->PDF->Multipage = UDC::MM_MULTI; itfProfile->OutputLocation->Mode = UDC::LM_PREDEFINED; itfProfile->OutputLocation->FolderPath = L"C:\\Out"; itfProfile->OutputLocation->FileName = L"&[DocName(0)].&[ImageType]"; itfProfile->OutputLocation->OverwriteExistingFile = FALSE; itfProfile->PostProcessing->Mode = UDC::PP_OPEN_FOLDER; // Run Microsoft Word as COM-server MSWORD::_ApplicationPtr itfWordApp(L"Word.Application"); MSWORD::_DocumentPtr itfWordDoc; // Open the document from a file itfWordDoc = itfWordApp->Documents->Open( COleVariant( szFilePath ) ); // Print all pages of the document itfWordApp->ActivePrinter = L"Universal Document Converter"; itfWordApp->PrintOut( vFalse ); // Close the document and Microsoft Word application itfWordDoc->Close( vFalse ); itfWordApp->Quit( vFalse, vFalse, vFalse ); }
Visual C#
C# Word to PDF conversion code example:
////////////////////////////////////////////////////////////////// // This example was designed to be used in Microsoft Visual C# from // Microsoft Visual Studio 2003 or higher. // // 1. Microsoft Word 97 or higher should be installed and activated on your PC. // // 2. Universal Document Converter 5.2 or higher should be installed as well. // // 3. Add references to Microsoft Word XX.0 Object Library and // Universal Document Converter Type Library using the // Project | Add Reference menu > COM tab. XX is the // Microsoft Office version installed on your computer. // // 4. Notice that the number of Microsoft Word's method parameters may depend on // the Office version. ////////////////////////////////////////////////////////////////// using System; using System.IO; using Word = Microsoft.Office.Interop.Word; //using Word; in VS2003 using UDC; namespace WordToPDF { class Program { static void PrintWordToPDF(string WordDocFilePath) { //Create a UDC object and get its interfaces IUDC objUDC = new APIWrapper(); IUDCPrinter Printer = objUDC.get_Printers("Universal Document Converter"); IProfile Profile = Printer.Profile; //Use Universal Document Converter API to change settings of //convertered document Profile.PageSetup.ResolutionX = 600; Profile.PageSetup.ResolutionY = 600; Profile.FileFormat.ActualFormat = FormatID.FMT_PDF; Profile.FileFormat.PDF.Multipage = MultipageModeID.MM_MULTI; Profile.OutputLocation.Mode = LocationModeID.LM_PREDEFINED; Profile.OutputLocation.FolderPath = @"c:\UDC Output Files"; Profile.OutputLocation.FileName = @"&[DocName(0)].&[ImageType]"; Profile.OutputLocation.OverwriteExistingFile = false; //Create a Word's Application object Word.Application WordApp = new Word.Application(); Object FilePath = WordDocFilePath; Object ReadOnly = true; //This will be passed when ever we don't want to pass value: Object Missing = Type.Missing; //Open document from file Word.Document WordDoc = WordApp.Documents.Open(ref FilePath, ref Missing, ref ReadOnly, ref Missing, ref Missing, ref Missing, ref Missing, ref Missing, ref Missing, ref Missing, ref Missing, ref Missing, ref Missing, ref Missing, ref Missing, ref Missing); //Print all pages of the document Object Background = false; WordApp.ActivePrinter = "Universal Document Converter"; WordApp.PrintOut(ref Background, ref Missing, ref Missing, ref Missing, ref Missing, ref Missing, ref Missing, ref Missing, ref Missing, ref Missing, ref Missing, ref Missing, ref Missing, ref Missing, ref Missing, ref Missing, ref Missing, ref Missing, ref Missing); //Close the document Object SaveChanges = false; WordDoc.Close(ref SaveChanges, ref Missing, ref Missing); //Quit the word application WordApp.Quit(ref Missing, ref Missing, ref Missing); } static void Main(string[] args) { string TestFilePath = Path.Combine(AppDomain.CurrentDomain.BaseDirectory, "TestFile.doc"); PrintWordToPDF(TestFilePath); } } }
Delphi
Delphi Word to PDF conversion code example:
////////////////////////////////////////////////////////////////// // This example was designed to be used in Delphi 7 or higher. // // 1. Microsoft Word 97 or higher should be installed and activated on your PC. // // 2. Universal Document Converter 5.2 or higher should be installed as well. // // 3. Add Universal Document Converter Type Library and // Microsoft Word XX.0 Object Library type libraries to the project. // XX is the Microsoft Office version installed on your computer. // // Delphi 7: // Use the Project | Import Type Library menu. // // Delphi 2006 or latter: // Use the Component | Import Component menu. // // Clear the Generate Component Wrapper checkbox and click the Create Unit // button (Delphi 7) or select the Create Unit option (Delphi 2006 or latter). // // 4. Notice that the number of Microsoft Word's method parameters may depend on // the Office version. // ////////////////////////////////////////////////////////////////// program WordToPDF; {$APPTYPE CONSOLE} {$DEFINE LATE_BINDING} uses SysUtils, Variants, Dialogs, ActiveX, Windows, Word_TLB, UDC_TLB; procedure PrintWordToPDF(WordDocFilePath: string); var objUDC: IUDC; Printer: IUDCPrinter; Profile: IProfile; WordApp: WordApplication; WordDoc: WordDocument; FilePath: OleVariant; ReadOnly: OleVariant; Missing: OleVariant; Background: OleVariant; SaveChanges: OleVariant; begin //Create a UDC object and get its interfaces objUDC := CoAPIWrapper.Create; Printer := objUDC.get_Printers('Universal Document Converter'); Profile := Printer.Profile; //Use Universal Document Converter API to change settings of convertered document Profile.PageSetup.ResolutionX := 600; Profile.PageSetup.ResolutionY := 600; Profile.FileFormat.ActualFormat := FMT_PDF; Profile.FileFormat.PDF.Multipage := MM_MULTI; Profile.OutputLocation.Mode := LM_PREDEFINED; Profile.OutputLocation.FolderPath := 'c:\UDC Output Files'; Profile.OutputLocation.FileName := '&[DocName(0)].&[ImageType]'; Profile.OutputLocation.OverwriteExistingFile := False; //Create a Word's Application object WordApp := CoWordApplication.Create; FilePath := WordDocFilePath; ReadOnly := True; //This will be passed when ever we don’t want to pass value: Missing := Variants.EmptyParam; //Open document from file {$IFDEF LATE_BINDING} WordDoc := IDispatch(OleVariant(WordApp).Documents.Open(FileName := FilePath, ReadOnly := ReadOnly)) as WordDocument; {$ELSE} WordDoc := WordApp.Documents.Open(FilePath, Missing, ReadOnly, Missing, Missing, Missing, Missing, Missing, Missing, Missing, Missing, Missing, Missing, Missing, Missing, Missing); {$ENDIF} //Print all pages of the document Background := False; WordApp.ActivePrinter := 'Universal Document Converter'; {$IFDEF LATE_BINDING} OleVariant(WordApp).PrintOut(Background := False); {$ELSE} WordApp.PrintOut(Background, Missing, Missing, Missing, Missing, Missing, Missing, Missing, Missing, Missing, Missing, Missing, Missing, Missing, Missing, Missing, Missing, Missing, Missing); {$ENDIF} //Close the document SaveChanges := False; {$IFDEF LATE_BINDING} OleVariant(WordDoc).Close(SaveChanges := SaveChanges); {$ELSE} WordDoc.Close(SaveChanges, Missing, Missing); {$ENDIF} //Quit the word application {$IFDEF LATE_BINDING} OleVariant(WordApp).Quit; {$ELSE} WordApp.Quit(Missing, Missing, Missing); {$ENDIF} end; var TestFilePath: string; begin TestFilePath := ExtractFilePath(ParamStr(0)) + 'TestFile.doc'; try CoInitialize(nil); try PrintWordToPDF(TestFilePath); finally CoUninitialize; end; except on E: Exception do MessageDlg(E.ClassName + ' : ' + E.Message, mtError, [mbOK], 0); end; end.
PHP
PHP Word to PDF converter code example:
'---------------------------------------------------------------------- ' 1) Microsoft Word 97 or above should be installed and activated on your PC. ' ' 2) Universal Document Converter 5.2 or above should also be installed. ' ' 3) Apache WEB server and PHP 4.0 or above should be installed and adjusted. '---------------------------------------------------------------------- <?PHP //Create Universal Document Converter object $objUDC = new COM("UDC.APIWrapper"); //Set up Universal Document Converter $itfPrinter = $objUDC->Printers("Universal Document Converter"); $itfProfile = $itfPrinter->Profile; $itfProfile->PageSetup->ResolutionX = 300; $itfProfile->PageSetup->ResolutionY = 300; $itfProfile->PageSetup->Orientation = 0; $itfProfile->FileFormat->ActualFormat = 7; //PDF $itfProfile->FileFormat->PDF->Multipage = 2; //Multipage mode $itfProfile->OutputLocation->Mode = 1; $itfProfile->OutputLocation->FolderPath = '&[Documents]\UDC Output Files\\'; $itfProfile->OutputLocation->FileName = '&[DocName(0)].&[ImageType]'; $itfProfile->OutputLocation->OverwriteExistingFile = 1; $itfProfile->PostProcessing->Mode = 0; //Create MS Word object $file = 'my_document.doc'; $WordApp = new COM("Word.Application"); //Print the document $WordDoc = $WordApp->Documents->Open($file,0,1); $WordApp->ActivePrinter = "Universal Document Converter"; $WordApp->PrintOut(False); //Close the document and MS Word $WordDoc->Close(); $WordApp->Quit(); echo "READY!"; ?>
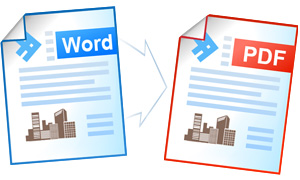