Automatic Conversion from PowerPoint to JPEG
Universal Document Converter works as a virtual printer and can save any document in the PDF, JPEG, TIFF, or PNG format. If you are a software developer, you can control the settings using COM-interface and using Microsoft PowerPoint as COM-server for converting your presentations to the JPEG format.
PowerPoint presentation conversion source code examples:
Visual Basic.NET
VB.NET PowerPoint to JPEG conversion code example:
'---------------------------------------------------------------------- ' 1) Microsoft PowerPoint starting from version 97 should be installed and ' activated on your PC. ' ' 2) Universal Document Converter version 5.2 or later should be installed ' as well. ' ' 3) Open your project in Microsoft Visual Basic.NET. ' ' 4) In Visual Basic main menu press Project->Add Reference... ' ' 5) In the Add Reference window go to COM tab and double click into ' Universal Document Converter Type Library '---------------------------------------------------------------------- Private Sub PrintPowerPointToJPEG(ByVal strFilePath As String) Dim objUDC As UDC.IUDC Dim itfPrinter As UDC.IUDCPrinter Dim itfProfile As UDC.IProfile Dim objPPTApp As Object Dim itfPresentation As Object Dim nSlides As Long objUDC = New UDC.APIWrapper itfPrinter = objUDC.Printers("Universal Document Converter") itfProfile = itfPrinter.Profile ' Use Universal Document Converter API to change settings of converterd document itfProfile.PageSetup.ResolutionX = 300 itfProfile.PageSetup.ResolutionY = 300 itfProfile.PageSetup.Orientation = UDC.PageOrientationID.PO_LANDSCAPE itfProfile.FileFormat.ActualFormat = UDC.FormatID.FMT_JPEG itfProfile.FileFormat.JPEG.ColorSpace = UDC.ColorSpaceID.CS_TRUECOLOR itfProfile.Adjustments.Crop.Mode = UDC.CropModeID.CRP_AUTO itfProfile.OutputLocation.Mode = UDC.LocationModeID.LM_PREDEFINED itfProfile.OutputLocation.FolderPath = "C:\Out" itfProfile.OutputLocation.FileName = "&[DocName(0)].&[ImageType]" itfProfile.OutputLocation.OverwriteExistingFile = False itfProfile.PostProcessing.Mode = UDC.PostProcessingModeID.PP_OPEN_FOLDER ' Run Microsoft PowerPoint as COM-server On Error Resume Next objPPTApp = CreateObject("PowerPoint.Application") ' Open document from file itfPresentation = objPPTApp.Presentations.Open(strFilePath, 1, 1, 0) ' Get number of slides in the presentation nSlides = itfPresentation.Slides.Count If nSlides > 0 Then ' Print all slides from the presentation itfPresentation.PrintOptions.PrintInBackground = 0 itfPresentation.PrintOptions.ActivePrinter = "Universal Document Converter" Call itfPresentation.PrintOut() End If ' Close the presentation Call itfPresentation.Close() itfPresentation = Nothing ' Close Microsoft PowerPoint Call objPPTApp.Quit() objPPTApp = Nothing End Sub
Visual Basic 6
VB PowerPoint to JPEG conversion code example:
'---------------------------------------------------------------------- ' 1) Microsoft PowerPoint 97 or higher should be installed and activated on your PC ' ' 2) Universal Document Converter 5.2 or higher should be installed as well ' ' 3) Open your project in Microsoft Visual Basic 6.0 ' ' 4) In Visual Basic main menu press Project->References ' ' 5) In the list of references check Universal Document Converter Type Library '---------------------------------------------------------------------- Private Sub PrintPowerPointToJPEG(strFilePath As String) Dim objUDC As IUDC Dim itfPrinter As IUDCPrinter Dim itfProfile As IProfile Dim objPPTApp As Object Dim itfPresentation As Object Set objUDC = New UDC.APIWrapper Set itfPrinter = objUDC.Printers("Universal Document Converter") Set itfProfile = itfPrinter.Profile ' Use Universal Document Converter API to change settings of converterd document itfProfile.PageSetup.ResolutionX = 300 itfProfile.PageSetup.ResolutionY = 300 itfProfile.PageSetup.Orientation = PO_LANDSCAPE itfProfile.FileFormat.ActualFormat = FMT_JPEG itfProfile.FileFormat.JPEG.ColorSpace = CS_TRUECOLOR itfProfile.Adjustments.Crop.Mode = CRP_AUTO itfProfile.OutputLocation.Mode = LM_PREDEFINED itfProfile.OutputLocation.FolderPath = "C:\Out" itfProfile.OutputLocation.FileName = "&[DocName(0)].&[ImageType]" itfProfile.OutputLocation.OverwriteExistingFile = False itfProfile.PostProcessing.Mode = PP_OPEN_FOLDER ' Run Microsoft PowerPoint as COM-server On Error Resume Next Set objPPTApp = CreateObject("PowerPoint.Application") ' Open document from file Err = 0 ' Clear GetLastError() value Set itfPresentation = objPPTApp.Presentations.Open(strFilePath, 1, 1, 0) If Err = 0 Then ' Get number of slides in the presentation nSlides = itfPresentation.Slides.Count If nSlides > 0 Then ' Print all slides from the presentation itfPresentation.PrintOptions.PrintInBackground = 0 itfPresentation.PrintOptions.ActivePrinter = "Universal Document Converter" Call itfPresentation.PrintOut End If ' Close the presentation Call itfPresentation.Close Set itfPresentation = Nothing End If ' Close Microsoft PowerPoint Call objPPTApp.Quit Set objPPTApp = Nothing End Sub
Visual C++
C++ PowerPoint to JPEG conversion code example:
////////////////////////////////////////////////////////////////// // This example was designed to be used in Microsoft Visual C++ starting // from Microsoft Visual Studio 2003 or higher. // // 1. Microsoft PowerPoint 97 or higher should be installed and activated on your PC. // // 2. Universal Document Converter 5.2 or higher should be installed as well. // // 3. You should initialize the COM before calling any COM method. // Please insert ::CoInitialize(0); in your application initialization // and ::CoUninitialize(); before closing it. // // 4. Import Office libraries for 32-bit version of Windows. // For 64-bit version please change C:\\Program Files\\ to // C:\\Program Files (x86)\\ in all pathes. #pragma message("Import MSO.DLL") // MS Office 2000 -> // "C:\\Program Files\\Common Files\\Microsoft Shared\\OFFICE10\\MSO.DLL" // // MS Office 2003 -> // "C:\\Program Files\\Common Files\\Microsoft Shared\\OFFICE11\\MSO.DLL" // // MS Office 2007 -> // "C:\\Program Files\\Common Files\\Microsoft Shared\\OFFICE12\\MSO.DLL" #import "C:\\Program Files\\Common Files\\Microsoft Shared\\OFFICE12\\MSO.DLL" \ rename_namespace("MSO"), auto_rename #pragma message("Import VBE6EXT.OLB") #import "C:\\Program Files\\Common Files\\Microsoft Shared\\VBA\\VBA6\\VBE6EXT.OLB" \ rename_namespace("VBE6EXT") #pragma message("Import MS Powerpoint API") // MS Office 2000 -> "C:\\Program Files\\Microsoft Office\\OFFICE\\MSPPT9.OLB" // MS Office 2003 -> "C:\\Program Files\\Microsoft Office\\OFFICE11\\MSPPT.OLB" // MS Office 2007 -> "C:\\Program Files\\Microsoft Office\\OFFICE12\\MSPPT.OLB" #import "C:\\Program Files\\Microsoft Office\\OFFICE12\\MSPPT.OLB"\ rename_namespace("POWERPNT"), auto_rename // 5. Import Universal Document Converter software API: #import "progid:udc.apiwrapper" rename_namespace("UDC") ////////////////////////////////////////////////////////////////// void PrintPowerPointToJPEG( CString sFilePath ) { UDC::IUDCPtr pUDC(__uuidof(UDC::APIWrapper)); UDC::IUDCPrinterPtr itfPrinter = pUDC->Printers["Universal Document Converter"]; UDC::IProfilePtr itfProfile = itfPrinter->Profile; // Use Universal Document Converter API to change settings of converterd document itfProfile->PageSetup->Orientation = UDC::PO_LANDSCAPE; itfProfile->FileFormat->ActualFormat = UDC::FMT_JPEG; itfProfile->FileFormat->JPEG->ColorSpace = UDC::CS_TRUECOLOR; itfProfile->OutputLocation->Mode = UDC::LM_PREDEFINED; itfProfile->OutputLocation->FolderPath = L"C:\\Out"; itfProfile->OutputLocation->FileName = L"&[DocName(0)].&[ImageType]"; itfProfile->OutputLocation->OverwriteExistingFile = FALSE; itfProfile->PostProcessing->Mode = UDC::PP_OPEN_FOLDER; // Run Microsoft Excel as COM-server POWERPNT::_ApplicationPtr objPPTApp(L"PowerPoint.Application"); POWERPNT::_PresentationPtr itfPresentation; POWERPNT::PrintOptionsPtr itfPrintOptions; // Open document from file itfPresentation = objPPTApp->Presentations->Open( (LPCTSTR)sFilePath, MSO::msoTrue, MSO::msoTrue, MSO::msoFalse ); // Print all slides from the presentation itfPrintOptions = itfPresentation->PrintOptions; itfPrintOptions->put_PrintInBackground( MSO::msoFalse ); itfPrintOptions->ActivePrinter = "Universal Document Converter"; itfPresentation->PrintOut( 0, itfPresentation->Slides->Count, _T(""), 1, MSO::msoFalse ); // Close the presentation itfPresentation->Close(); // Close Microsoft PowerPoint objPPTApp->Quit(); }
Visual C#
C# PowerPoint to JPEG conversion code example:
/////////////////////////////////////////////////////////////////////////////////// // This example was designed to be used in Microsoft Visual C# starting // from Microsoft Visual Studio 2003 or higher. // // 1. Microsoft PowerPoint 97 or higher should be installed and activated on your PC // // 2. Universal Document Converter 5.2 or higher should be installed as well // // 3. Add references to Microsoft PowerPoint XX.0 Object Library and // Universal Document Converter Type Library // using the Project | Add Reference menu > COM tab. // XX is the Microsoft Office version installed on your computer. /////////////////////////////////////////////////////////////////////////////////// using System; using System.IO; using UDC; using PowerPoint = Microsoft.Office.Interop.PowerPoint; //using PowerPoint; in VS2003 using MSO = Microsoft.Office.Core; namespace PowerPointToJPEG { class Program { static void PowerPointToJPEG(string pptFilePath) { //Create a UDC object and get its interfaces IUDC objUDC = new APIWrapper(); IUDCPrinter Printer = objUDC.get_Printers("Universal Document Converter"); IProfile Profile = Printer.Profile; //Use Universal Document Converter API to change settings of //converterd document Profile.PageSetup.Orientation = PageOrientationID.PO_LANDSCAPE; Profile.FileFormat.ActualFormat = FormatID.FMT_JPEG; Profile.FileFormat.JPEG.ColorSpace = ColorSpaceID.CS_TRUECOLOR; Profile.OutputLocation.Mode = LocationModeID.LM_PREDEFINED; Profile.OutputLocation.FolderPath = @"c:\UDC Output Files"; Profile.OutputLocation.FileName = @"&[DocName(0)].&[ImageType]"; Profile.OutputLocation.OverwriteExistingFile = false; Profile.PostProcessing.Mode = PostProcessingModeID.PP_OPEN_FOLDER; //Run Microsoft Excel as COM-server PowerPoint.Application PowerPointApp = new PowerPoint.ApplicationClass(); //Open document from file PowerPoint.Presentation Presentation = PowerPointApp.Presentations.Open(pptFilePath, MSO.MsoTriState.msoTrue, MSO.MsoTriState.msoTrue, MSO.MsoTriState.msoFalse); //Print all slides from the presentation PowerPoint.PrintOptions PrintOptions = Presentation.PrintOptions; PrintOptions.PrintInBackground = MSO.MsoTriState.msoFalse; PrintOptions.ActivePrinter = "Universal Document Converter"; Presentation.PrintOut(0, Presentation.Slides.Count, "", 1, MSO.MsoTriState.msoFalse); //Close the presentation Presentation.Close(); //Close Microsoft PowerPoint PowerPointApp.Quit(); } static void Main(string[] args) { string TestFilePath = Path.Combine(AppDomain.CurrentDomain.BaseDirectory, "TestFile.ppt"); PowerPointToJPEG(TestFilePath); } } }
Delphi
Delphi PowerPoint to JPEG conversion code example:
/////////////////////////////////////////////////////////////////////////////////// // This example was designed to be used in Delphi - version 7 or higher. // // 1. Microsoft PowerPoint 97 or higher should be installed and activated on your PC // // 2. Universal Document Converter 5.2 or higher should be installed as well // // 3. Add UDC Type Library and Microsoft PowerPoint XX.0 Object Library type // libraries to the project. XX is the Microsoft Office version installed on your PC // // Delphi 7: // Use the Project | Import Type Library menu. // // Delphi 2006 or latter: // Use the Component | Import Component menu. // // Clear the Generate Component Wrapper checkbox and click the Create Unit // button (Delphi 7) or select the Create Unit option (Delphi 2006 or latter). // // 4. Notice that the number of Microsoft PowerPoint's method parameters may depend on // the Microsoft Office version you have. /////////////////////////////////////////////////////////////////////////////////// program PowerPointToJPEG; {$APPTYPE CONSOLE} uses SysUtils, Variants, Dialogs, ActiveX, Windows, UDC_TLB, Office_TLB, PowerPoint_TLB; procedure PrintPowerPointToJPEG(pptFilePath: string); var objUDC: IUDC; Printer: IUDCPrinter; Profile: IProfile; PowerPointApp: PowerPointApplication; Presentation: PowerPointPresentation; PrintOptions: PowerPoint_TLB.PrintOptions; begin //Create a UDC object and get its interfaces objUDC := CoAPIWrapper.Create; Printer := objUDC.get_Printers('Universal Document Converter'); Profile := Printer.Profile; //Use Universal Document Converter API to change settings of converterd document Profile.PageSetup.Orientation := PO_LANDSCAPE; Profile.FileFormat.ActualFormat := FMT_JPEG; Profile.FileFormat.JPEG.ColorSpace := CS_TRUECOLOR; Profile.OutputLocation.Mode := LM_PREDEFINED; Profile.OutputLocation.FolderPath := 'c:\UDC Output Files'; Profile.OutputLocation.FileName := '&[DocName(0)].&[ImageType]'; Profile.OutputLocation.OverwriteExistingFile := False; Profile.PostProcessing.Mode := PP_OPEN_FOLDER; //Run Microsoft Excel as COM-server PowerPointApp := CoPowerPointApplication.Create; //Open document from file Presentation := PowerPointApp.Presentations.Open(pptFilePath, msoTrue, msoTrue, msoFalse); //Print all slides from the presentation PrintOptions := Presentation.PrintOptions; PrintOptions.PrintInBackground := msoFalse; PrintOptions.ActivePrinter := 'Universal Document Converter'; Presentation.PrintOut(0, Presentation.Slides.Count, '', 1, msoFalse); //Close the presentation Presentation.Close(); //Close Microsoft PowerPoint PowerPointApp.Quit(); end; var TestFilePath: string; begin TestFilePath := ExtractFilePath(ParamStr(0)) + 'TestFile.ppt'; try CoInitialize(nil); try PrintPowerPointToJPEG(TestFilePath); finally CoUninitialize; end; except on E: Exception do MessageDlg(E.ClassName + ' : ' + E.Message, mtError, [mbOK], 0); end; end.
PHP
PHP PowerPoint to JPEG conversion code example:
'---------------------------------------------------------------------- ' 1) Microsoft Power Point 97 or above should be installed and activated on your PC. ' ' 2) Universal Document Converter 5.2 or above should also be installed. ' ' 3) Apache WEB server and PHP 4.0 or above should be installed and adjusted. '---------------------------------------------------------------------- <?PHP //Create Universal Document Converter object $objUDC = new COM("UDC.APIWrapper"); //Set up Universal Document Converter $itfPrinter = $objUDC->Printers("Universal Document Converter"); $itfProfile = $itfPrinter->Profile; $itfProfile->PageSetup->ResolutionX = 300; $itfProfile->PageSetup->ResolutionY = 300; $itfProfile->PageSetup->Orientation = 0; $itfProfile->PageSetup->Units = 1; $itfProfile->PageSetup->Width = 220; $itfProfile->PageSetup->Height = 180; $itfProfile->FileFormat->ActualFormat = 2; $itfProfile->FileFormat->JPEG->ColorSpace = 24; $itfProfile->FileFormat->JPEG->Mode = 0; $itfProfile->FileFormat->JPEG->Quality = 50; $itfProfile->OutputLocation->Mode = 1; $itfProfile->OutputLocation->FolderPath = '&[Documents]\UDC Output Files\\'; $itfProfile->OutputLocation->FileName = '&[DocName(0)].&[ImageType]'; $itfProfile->OutputLocation->OverwriteExistingFile = 0; $itfProfile->Adjustments->Crop->Mode = 0; $itfProfile->PostProcessing->Mode = 0; //Create MS Power Point object and open the file $file = 'my_presentation.ppt'; $PPTApp = new COM("PowerPoint.Application"); $Presentation = $PPTApp->Presentations->Open($file,1,1,0); //Print setup $Presentation->PrintOptions->PrintInBackground = 0; $Presentation->PrintOptions->ActivePrinter = "Universal Document Converter"; //Print the presentation $Presentation->PrintOut; //Close the document $Presentation->Close; //Close Power Point $PPTApp->Quit; echo "READY!"; ?>
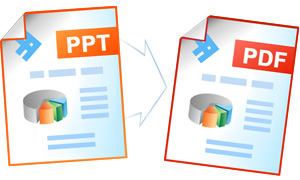