Excel to PDF Conversion Automation
Converting documents into image files or into the PDF format is a pretty frequent necessity. Universal Document Converter can help you process documents converting them into PDF, JPEG, PNG, or TIFF formats.
The “conversion kernel” of the Universal Document Converter can be utilized by software developers for their own projects with similar conversion tasks by using COM-interface and Microsoft Excel as COM-server for converting Excel to PDF.
Excel worksheet conversion source code examples:
Visual Basic.NET
VB.NET Excel to PDF conversion code example:
'---------------------------------------------------------------------- ' 1) Microsoft Excel version 97 or higher should be installed and ' activated on your PC ' ' 2) Universal Document Converter 5.2 or higher should be installed as well ' ' 3) Open your project in Microsoft Visual Basic.NET ' ' 4) In Visual Basic main menu press Project->Add Reference... ' ' 5) In the Add Reference window go to COM tab and double click into ' Universal Document Converter Type Library ' ' 6) Before using this example, please read this article from ' Microsoft Excel 2003 knowledge base: ' http://support.microsoft.com/kb/320369/en-us/ '---------------------------------------------------------------------- Private Sub PrintExcelToPDF(ByVal strFilePath As String) Dim objUDC As UDC.IUDC Dim itfPrinter As UDC.IUDCPrinter Dim itfProfile As UDC.IProfile Dim objXLApp As Object Dim itfXLBook As Object Dim itfXLWorksheet As Object Dim itfXLPageSetup As Object objUDC = New UDC.APIWrapper itfPrinter = objUDC.Printers("Universal Document Converter") itfProfile = itfPrinter.Profile ' Use Universal Document Converter API to change settings of converterd document itfProfile.PageSetup.FormName = "A2" itfProfile.PageSetup.ResolutionX = 200 itfProfile.PageSetup.ResolutionY = 200 itfProfile.PageSetup.Orientation = UDC.PageOrientationID.PO_LANDSCAPE itfProfile.FileFormat.ActualFormat = UDC.FormatID.FMT_PDF itfProfile.FileFormat.PDF.Multipage = UDC.MultipageModeID.MM_MULTI itfProfile.Adjustments.Crop.Mode = UDC.CropModeID.CRP_AUTO itfProfile.OutputLocation.Mode = UDC.LocationModeID.LM_PREDEFINED itfProfile.OutputLocation.FolderPath = "C:\Out" itfProfile.OutputLocation.FileName = "&[DocName(0)].&[ImageType]" itfProfile.OutputLocation.OverwriteExistingFile = False ' Run Microsoft Excle as COM-server On Error Resume Next objXLApp = CreateObject("Excel.Application") ' Open spreadsheet from file itfXLBook = objXLApp.Workbooks.Open(strFilePath, , True) ' Change active worksheet settings and print it itfXLWorksheet = itfXLBook.ActiveSheet itfXLPageSetup = itfXLWorksheet.PageSetup itfXLPageSetup.Orientation = 2 ' Landscape Call itfXLWorksheet.PrintOut(, , , False, "Universal Document Converter") ' Close the spreadsheet Call itfXLBook.Close(False) itfXLBook = Nothing ' Close Microsoft Excel Call objXLApp.Quit() objXLApp = Nothing End Sub
Visual Basic 6
VB Excel to PDF conversion code example:
'---------------------------------------------------------------------- ' 1) Microsoft Excel version 97 or higher should be installed and ' activated on your PC ' ' 2) Universal Document Converter 5.2 or higher should be installed as well ' ' 3) Open your project in Microsoft Visual Basic 6.0 ' ' 4) In Visual Basic main menu press Project->References ' ' 5) In the list of references check Universal Document Converter Type Library ' ' 6) Before using this example, please read this article from ' Microsoft Excel 2003 knowledge base: ' http://support.microsoft.com/kb/320369/en-us/ '---------------------------------------------------------------------- Private Sub PrintExcelToPDF(strFilePath As String) Dim objUDC As IUDC Dim itfPrinter As IUDCPrinter Dim itfProfile As IProfile Dim ExcelApp As Object Dim ExcelBook As Object Dim ExcelWorksheet As Object Dim ExcelPageSetup As Object Set objUDC = New UDC.APIWrapper Set itfPrinter = objUDC.Printers("Universal Document Converter") Set itfProfile = itfPrinter.Profile ' Use Universal Document Converter API to change settings of converterd document itfProfile.PageSetup.FormName = "A2" itfProfile.PageSetup.ResolutionX = 200 itfProfile.PageSetup.ResolutionY = 200 itfProfile.PageSetup.Orientation = PO_LANDSCAPE itfProfile.FileFormat.ActualFormat = FMT_PDF itfProfile.FileFormat.PDF.Multipage = MM_MULTI itfProfile.Adjustments.Crop.Mode = CRP_AUTO itfProfile.OutputLocation.Mode = LM_PREDEFINED itfProfile.OutputLocation.FolderPath = "C:\Out" itfProfile.OutputLocation.FileName = "&[DocName(0)].&[ImageType]" itfProfile.OutputLocation.OverwriteExistingFile = False ' Run Microsoft Excle as COM-server On Error Resume Next Set ExcelApp = CreateObject("Excel.Application") ' Open spreadsheet from file Err = 0 ' Clear GetLastError() value Set ExcelBook = ExcelApp.Workbooks.Open(strFilePath, , True) If Err = 0 Then ' Change active worksheet settings and print it Set ExcelWorksheet = ExcelBook.ActiveSheet Set ExcelPageSetup = ExcelWorksheet.PageSetup ExcelPageSetup.Orientation = 2 ' Landscape Call ExcelWorksheet.PrintOut(, , , False, "Universal Document Converter") ' Close the spreadsheet Call ExcelBook.Close(False) Set ExcelBook = Nothing End If ' Close Microsoft Excel Call ExcelApp.Quit Set ExcelApp = Nothing End Sub
Visual C++
C++ Excel to PDF conversion code example:
////////////////////////////////////////////////////////////////// // This example was designed to be used in Microsoft Visual C++ from // Microsoft Visual Studio version 2003 or higher // // 1. Microsoft Excel version 97 or higher should be installed and // activated on your PC // // 2. Before using this example, please read this article from // Microsoft Excel 2003 knowledge base: // http://support.microsoft.com/kb/320369/en-us/ // // 3. Universal Document Converter 5.2 or higher should be installed as well // // 4. You must initialize the COM before you call any COM method. // Please insert ::CoInitialize(0); in your application initialization // and ::CoUninitialize(); before closing it. // // 5. Import Office libraries for 32-bit version of Windows. // For 64-bit version please change C:\\Program Files\\ to // C:\\Program Files (x86)\\ in all pathes. #pragma message("Import MSO.DLL") // MS Office 2000 -> // "C:\\Program Files\\Common Files\\Microsoft Shared\\OFFICE10\\MSO.DLL" // // MS Office 2003 -> // "C:\\Program Files\\Common Files\\Microsoft Shared\\OFFICE11\\MSO.DLL" // // MS Office 2007 -> // "C:\\Program Files\\Common Files\\Microsoft Shared\\OFFICE12\\MSO.DLL" #import "C:\\Program Files\\Common Files\\Microsoft Shared\\OFFICE12\\MSO.DLL" \ rename_namespace("MSO"), auto_rename #pragma message("Import VBE6EXT.OLB") #import "C:\\Program Files\\Common Files\\Microsoft Shared\\VBA\\VBA6\\VBE6EXT.OLB" \ rename_namespace("VBE6EXT") #pragma message("Import MS Excel API") // MS Office 2000 -> "C:\\Program Files\\Microsoft Office\\OFFICE\\Excel9.OLB" // MS Office 2003 -> "C:\\Program Files\\Microsoft Office\\OFFICE11\\Excel.EXE" // MS Office 2007 -> "C:\\Program Files\\Microsoft Office\\OFFICE12\\Excel.EXE" #import "C:\\Program Files\\Microsoft Office\\OFFICE12\\Excel.EXE"\ rename_namespace("MSEXCEL"), auto_rename // 6. Import Universal Document Converter software API: #import "progid:udc.apiwrapper" rename_namespace("UDC") ////////////////////////////////////////////////////////////////// static COleVariant vTrue( (short)TRUE ), vFalse( (short)FALSE ); static COleVariant vOpt( (long)DISP_E_PARAMNOTFOUND, VT_ERROR ); void PrintExcelToPDF( CString sFilePath ) { UDC::IUDCPtr pUDC(__uuidof(UDC::APIWrapper)); UDC::IUDCPrinterPtr itfPrinter = pUDC->Printers["Universal Document Converter"]; UDC::IProfilePtr itfProfile = itfPrinter->Profile; // Use Universal Document Converter API to change settings of converterd document itfProfile->PageSetup->ResolutionX = 600; itfProfile->PageSetup->ResolutionY = 600; itfProfile->FileFormat->ActualFormat = UDC::FMT_PDF; itfProfile->FileFormat->PDF->Multipage = UDC::MM_MULTI; itfProfile->OutputLocation->Mode = UDC::LM_PREDEFINED; itfProfile->OutputLocation->FolderPath = L"C:\\Out"; itfProfile->OutputLocation->FileName = L"&[DocName(0)].&[ImageType]"; itfProfile->OutputLocation->OverwriteExistingFile = FALSE; itfProfile->PostProcessing->Mode = UDC::PP_OPEN_FOLDER; // Run Microsoft Excel as COM-server MSEXCEL::_ApplicationPtr itfXLApp(L"Excel.Application"); MSEXCEL::_WorkbookPtr itfXLBook; MSEXCEL::_WorksheetPtr itfXLWorksheet; MSEXCEL::PageSetupPtr itfXLPageSetup; // Open the document from a file itfXLBook = itfXLApp->Workbooks->Open( (LPCTSTR)sFilePath, vOpt, vTrue ); // Change active worksheet settings and print it itfXLWorksheet = itfXLBook->ActiveSheet; itfXLPageSetup = itfXLWorksheet->PageSetup; itfXLPageSetup->Orientation = MSEXCEL::xlLandscape; itfXLWorksheet->PrintOut( vOpt, vOpt, vOpt, vFalse, "Universal Document Converter"); // Close the spreadsheet without saving changes itfXLBook->Close( vFalse ); // Close Microsoft Excel itfXLApp->Quit(); }
Visual C#
C# Excel to PDF conversion code example:
////////////////////////////////////////////////////////////////// // This example was designed to be used in Microsoft Visual C# from // Microsoft Visual Studio version 2003 or higher. // // 1. Microsoft Excel version 97 or higher should be installed and activated // on your PC. // // 2. Before using this example, please read this article from // Microsoft Excel 2003 knowledge base: // http://support.microsoft.com/kb/320369/en-us/ // A workaround for this issue is available in this example. // // 3. Universal Document Converter version 5.2 or higher should be installed as well. // // 4. Add references to Microsoft Excel XX.0 Object Library // and Universal Document Converter Type Library using the // Project | Add Reference menu > COM tab. // XX is the Microsoft Office version installed on your computer. ////////////////////////////////////////////////////////////////// using System; using System.IO; using UDC; using Excel = Microsoft.Office.Interop.Excel; //using Excel; in VS2003 namespace ExcelToPDF { class Program { static void PrintExcelToPDF(string xlFilePath) { //Create a UDC object and get its interfaces IUDC objUDC = new APIWrapper(); IUDCPrinter Printer = objUDC.get_Printers("Universal Document Converter"); IProfile Profile = Printer.Profile; //Use Universal Document Converter API to change settings of //converterd document Profile.PageSetup.ResolutionX = 600; Profile.PageSetup.ResolutionY = 600; Profile.FileFormat.ActualFormat = FormatID.FMT_PDF; Profile.FileFormat.PDF.Multipage = MultipageModeID.MM_MULTI; Profile.OutputLocation.Mode = LocationModeID.LM_PREDEFINED; Profile.OutputLocation.FolderPath = @"c:\UDC Output Files"; Profile.OutputLocation.FileName = @"&[DocName(0)].&[ImageType]"; Profile.OutputLocation.OverwriteExistingFile = false; Profile.PostProcessing.Mode = PostProcessingModeID.PP_OPEN_FOLDER; //Create a Excel's Application object Excel.Application ExcelApp = new Excel.ApplicationClass(); Object ReadOnly = true; //This will be passed when ever we don't want to pass value: Object Missing = Type.Missing; //If you run an English version of Excel on a computer with the regional //settings are configured for a non-English language, you must set the //CultureInfo prior calling Excel methods. System.Threading.Thread.CurrentThread.CurrentCulture = new System.Globalization.CultureInfo("en-US"); //Open the document from a file Excel.Workbook Workbook = ExcelApp.Workbooks.Open(xlFilePath, Missing, ReadOnly, Missing, Missing, Missing, Missing, Missing, Missing, Missing, Missing, Missing, Missing, Missing, Missing); //Change active worksheet settings and print it Excel.Worksheet Worksheet = (Excel.Worksheet)Workbook.ActiveSheet; Excel.PageSetup PageSetup = Worksheet.PageSetup; PageSetup.Orientation = Excel.XlPageOrientation.xlLandscape; Object Preview = false; Worksheet.PrintOut(Missing, Missing, Missing, Preview, "Universal Document Converter", Missing, Missing, Missing); //Close the spreadsheet without saving changes Object SaveChanges = false; Workbook.Close(SaveChanges, Missing, Missing); //Close Microsoft Excel ExcelApp.Quit(); } static void Main(string[] args) { string TestFilePath = Path.Combine(AppDomain.CurrentDomain.BaseDirectory, "TestFile.xls"); PrintExcelToPDF(TestFilePath); } } }
Delphi
Delphi Excel to PDF conversion code example:
//////////////////////////////////////////////////////////////////////////////////// // This example was designed to be used in Delphi version 7 or higher // // 1. Microsoft Excel version 97 or higher should be installed and // activated on your PC // // 2. Universal Document Converter version 5.2 or higher should be installed as well // // 3. Before using this example, please read this article from // Microsoft Excel 2003 knowledge base: // http://support.microsoft.com/kb/320369/en-us/ // A workaround for this issue is available in this example. // // 4. Add Universal Document Converter Type Library and // Microsoft Excel XX.0 Object Library type libraries to the project. // XX is the Microsoft Office version installed on your computer. // // Delphi 7: // Use the Project | Import Type Library menu. // // Delphi 2006 or latter: // Use the Component | Import Component menu. // // Clear the Generate Component Wrapper checkbox and click the Create Unit // button (Delphi 7) or select the Create Unit option (Delphi 2006 or latter). // // 5. Notice that the number of Microsoft Excel's method parameters may depend // on the Office version. // //////////////////////////////////////////////////////////////////////////////////// program ExcelToPDF; {$APPTYPE CONSOLE} {$DEFINE LATE_BINDING} uses SysUtils, Variants, Windows, Dialogs, ActiveX, UDC_TLB, Excel_TLB; procedure PrintExcelToPDF(xlFilePath: string); var objUDC: IUDC; Printer: IUDCPrinter; Profile: IProfile; ExcelApp: ExcelApplication; Workbook: ExcelWorkbook; Worksheet: ExcelWorksheet; PageSetup: Excel_TLB.PageSetup; ReadOnly: OleVariant; Missing: OleVariant; Preview: OleVariant; SaveChanges: OleVariant; {$IFNDEF LATE_BINDING} LocaleID: Integer; {$ENDIF} begin //Create a UDC object and get its interfaces objUDC := CoAPIWrapper.Create; Printer := objUDC.get_Printers('Universal Document Converter'); Profile := Printer.Profile; //Use Universal Document Converter API to change settings of converterd document Profile.PageSetup.ResolutionX := 150; Profile.PageSetup.ResolutionY := 150; Profile.FileFormat.ActualFormat := FMT_PDF; Profile.FileFormat.PDF.Multipage := MM_MULTI; Profile.OutputLocation.Mode := LM_PREDEFINED; Profile.OutputLocation.FolderPath := 'c:\UDC Output Files'; Profile.OutputLocation.FileName := '&[DocName(0)].&[ImageType]'; Profile.OutputLocation.OverwriteExistingFile := False; Profile.PostProcessing.Mode := PP_OPEN_FOLDER; //Create a Excel's Application object ExcelApp := CoExcelApplication.Create; ReadOnly := True; //This will be passed when ever we don't want to pass value Missing := Variants.EmptyParam; //If you run an English version of Excel on a computer with the regional settings //are configured for a non-English language, you must pass the correct locale ID. //Open the document from a file {$IFDEF LATE_BINDING} Workbook := IDispatch(OleVariant(ExcelApp).Workbooks.Open(FileName := xlFilePath, ReadOnly := ReadOnly)) as ExcelWorkbook; {$ELSE} LocaleID := $0409; //set en-US locale or call GetThreadLocale Workbook := ExcelApp.Workbooks.Open(xlFilePath, Missing, ReadOnly, Missing, Missing, Missing, Missing, Missing, Missing, Missing, Missing, Missing, Missing, Missing, Missing, LocaleID); {$ENDIF} //Change active worksheet settings and print it Worksheet := Workbook.ActiveSheet as ExcelWorksheet; PageSetup := Worksheet.PageSetup; PageSetup.Orientation := xlLandscape; Preview := False; {$IFDEF LATE_BINDING} OleVariant(Worksheet).PrintOut(Preview := Preview, ActivePrinter := 'Universal Document Converter'); {$ELSE} Worksheet.PrintOut(Missing, Missing, Missing, Preview, 'Universal Document Converter', Missing, Missing, Missing, LocaleID); {$ENDIF} //Close the spreadsheet without saving changes SaveChanges := False; {$IFDEF LATE_BINDING} OleVariant(Workbook).Close(SaveChanges := SaveChanges); {$ELSE} Workbook.Close(SaveChanges, Missing, Missing, LocaleID); {$ENDIF} //Close Microsoft Excel ExcelApp.Quit(); end; var TestFilePath: string; begin TestFilePath := ExtractFilePath(ParamStr(0)) + 'TestFile.xls'; try CoInitialize(nil); try PrintExcelToPDF(TestFilePath); finally CoUninitialize; end; except on E: Exception do MessageDlg(E.ClassName + ' : ' + E.Message, mtError, [mbOK], 0); end; end.
PHP
PHP Excel to PDF converter code example:
'---------------------------------------------------------------------- ' 1) Microsoft Excel 97 or above should be installed and activated on your PC ' ' 2) Universal Document Converter 5.2 or above should also be installed ' ' 3) Apache WEB server and PHP 4.0 or above should be installed and adjusted. '---------------------------------------------------------------------- <?PHP //Create Universal Document Converter object $objUDC = new COM("UDC.APIWrapper"); //Set up Universal Document Converter $itfPrinter = $objUDC->Printers("Universal Document Converter"); $itfProfile = $itfPrinter->Profile; $itfProfile->PageSetup->ResolutionX = 300; $itfProfile->PageSetup->ResolutionY = 300; $itfProfile->PageSetup->Orientation = 0; $itfProfile->FileFormat->ActualFormat = 7; //PDF $itfProfile->FileFormat->PDF->Multipage = 2; //Multipage mode $itfProfile->OutputLocation->Mode = 1; $itfProfile->OutputLocation->FolderPath = '&[Documents]\UDC Output Files\\'; $itfProfile->OutputLocation->FileName = '&[DocName(0)].&[ImageType]'; $itfProfile->OutputLocation->OverwriteExistingFile = 1; $itfProfile->PostProcessing->Mode = 0; //Create MS Excel object and open the file $file = 'my_file.xls'; $ExcelApp = new COM("Excel.Application"); $ExcelBook = $ExcelApp->Workbooks->Open($file,0,1); //change the orientation of the active worcksheet $ExcelWorksheet = $ExcelBook->ActiveSheet; $ExcelPageSetup = $ExcelWorksheet->PageSetup; $ExcelPageSetup->Orientation = 2; //Printing the curent sheet $ExcelWorksheet->PrintOut(1,1,1,False,"Universal Document Converter"); //Close the document $ExcelBook->Close(False); //Close Excel $ExcelApp->Quit; echo "READY!"; ?>
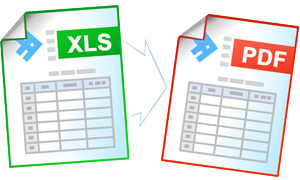