Visio to TIFF Conversion Automation
Universal Document Converter is the software that can convert any document into PDF, JPEG, TIFF, or PNG files. Software developers can utilize the Universal Document Converter settings and apply them to be used with the COM-interface with the Microsoft Visio as the COM-server. This will allow converting drawings into TIFF files.
Visio drawing conversion source code examples:
Visual Basic.NET
VB.NET Visio to TIFF conversion code example:
'---------------------------------------------------------------------- ' 1) Microsoft Visio version 2000 or higher should be installed and activated ' on your PC ' ' 2) Universal Document Converter 5.2 or higher should be installed as well ' ' 3) Open your project in Microsoft Visual Basic.NET. ' ' 4) In Visual Basic main menu press Project->Add Reference... ' ' 5) In Add Reference window go to COM tab and double click on the ' Universal Document Converter Type Library '---------------------------------------------------------------------- Private Sub PrintVisioToTIFF(ByVal strFilePath As String) ' Define constants Const visOpenRW = &H20 Const visOpenDontList = &H8 Const visPrintAll = 0 Dim objUDC As UDC.IUDC Dim itfPrinter As UDC.IUDCPrinter Dim itfProfile As UDC.IProfile Dim objVisioApp As Object Dim itfDrawing As Object Dim AppDataPath As String Dim ProfilePath As String ' Use Universal Document Converter API to change settings of converterd document objUDC = New UDC.APIWrapper itfPrinter = objUDC.Printers("Universal Document Converter") itfProfile = itfPrinter.Profile ' Load profile located in folder "%APPDATA%\UDC Profiles". ' Value of %APPDATA% variable should be received using Environment.GetFolderPath ' method. Or you can move default profiles into a folder you prefer. AppDataPath = Environment.GetFolderPath(Environment.SpecialFolder.ApplicationData) ProfilePath = Path.Combine(AppDataPath, "UDC Profiles\Drawing to TIFF.xml") itfProfile.Load(ProfilePath) itfProfile.OutputLocation.Mode = UDC.LocationModeID.LM_PREDEFINED itfProfile.OutputLocation.FolderPath = "C:\Out" itfProfile.PostProcessing.Mode = UDC.PostProcessingModeID.PP_OPEN_FOLDER ' Run Microsoft Visio as COM-server On Error Resume Next objVisioApp = CreateObject("Visio.Application") objVisioApp.Visible = False ' Open drawing from file itfDrawing = objVisioApp.Documents.OpenEx(strFilePath, visOpenRW And visOpenDontList) ' Change Drawing preferences for scaling it to page itfDrawing.PrintCenteredH = True itfDrawing.PrintCenteredV = True itfDrawing.PrintFitOnPages = True ' Print drawing itfDrawing.Printer = "Universal Document Converter" itfDrawing.PrintOut(visPrintAll) ' Close drawing itfDrawing.Saved = True itfDrawing.Close() itfDrawing = Nothing ' Close Microsoft Visio Call objVisioApp.Quit() objVisioApp = Nothing End Sub
Visual Basic 6
VB Visio to TIFF conversion code example:
'---------------------------------------------------------------------- ' 1) Microsoft Visio version 2000 or higher should be installed and activated ' on your PC ' ' 2) Universal Document Converter version 5.2 or higher should be installed as well ' ' 3) Open your project in Microsoft Visual Basic 6.0 ' ' 4) In Visual Basic main menu press Project->References ' ' 5) In the list of references check Universal Document Converter Type Library '---------------------------------------------------------------------- Private Sub PrintVisioToTIFF(strFilePath As String) ' Define constants Const visOpenRW = &H20 Const visOpenDontList = &H8 Const visPrintAll = 0 Dim objUDC As IUDC Dim itfPrinter As IUDCPrinter Dim itfProfile As IProfile Dim objVisioApp As Object Dim itfDrawing As Object ' Use Universal Document Converter API to change settings of converterd document Set objUDC = New UDC.APIWrapper Set itfPrinter = objUDC.Printers("Universal Document Converter") Set itfProfile = itfPrinter.Profile ' Load profile located in folder "%APPDATA%\UDC Profiles". ' Value of %APPDATA% variable should be received using Windows API's ' SHGetSpecialFolderPath function. Or you can move default profiles into a folder ' you prefer. itfProfile.Load("Drawing to TIFF.xml") itfProfile.OutputLocation.Mode = LM_PREDEFINED itfProfile.OutputLocation.FolderPath = "C:\Out" itfProfile.PostProcessing.Mode = PP_OPEN_FOLDER ' Run Microsoft Visio as COM-server On Error Resume Next Set objVisioApp = CreateObject("Visio.Application") objVisioApp.Visible = False ' Open drawing from file Err = 0 ' Clear GetLastError() value Set itfDrawing = objVisioApp.Documents.OpenEx(strFilePath, visOpenRW And visOpenDontList) If Err = 0 Then ' Change Drawing preferences for scaling it to page itfDrawing.PrintCenteredH = True itfDrawing.PrintCenteredV = True itfDrawing.PrintFitOnPages = True ' Print drawing itfDrawing.Printer = "Universal Document Converter" itfDrawing.PrintOut (visPrintAll) ' Close drawing itfDrawing.Saved = True itfDrawing.Close Set itfDrawing = Nothing End If ' Close Microsoft Visio Call objVisioApp.Quit Set objVisioApp = Nothing End Sub
Visual C++
C++ Visio to TIFF conversion code example:
////////////////////////////////////////////////////////////////// // This example was designed to be used in Microsoft Visual C++ from // Microsoft Visual Studio version 2003 or higher // // 1. Microsoft Visio version 2002 or higher should be installed and activated // on your PC // // 2. Universal Document Converter 5.2 or higher should be installed as well // // 3. You must initialize the COM before you call any COM method. // Please insert ::CoInitialize(0); in your application initialization // and ::CoUninitialize(); before closing it. // // 4. Import Visio library for 32-bit version of Windows. // For 64-bit version please change C:\\Program Files\\ to // C:\\Program Files (x86)\\ in all paths. #pragma message("Import MS Visio API") // MS Visio 2002 -> "C:\\Program Files\\Microsoft Office\\Visio10\\Vislib.dll" // MS Visio 2003 -> "C:\\Program Files\\Microsoft Office\\Visio11\\VISLIB.DLL" // MS Visio 2007 -> "C:\\Program Files\\Microsoft Office\\OFFICE12\\VISLIB.DLL" #import "C:\\Program Files\\Microsoft Office\\OFFICE12\\VISLIB.DLL"\ rename_namespace("VISIO"), auto_rename // 5. Import Universal Document Converter software API: #import "progid:udc.apiwrapper" rename_namespace("UDC") ////////////////////////////////////////////////////////////////// void PrintVisioToTIFF( CString sFilePath ) { // Define constants const int visOpenDontList = 0x08, visOpenRW = 0x20; const int visOpenHidden = 64, visOpenMacrosDisabled = 128; UDC::IUDCPtr pUDC(__uuidof(UDC::APIWrapper)); UDC::IUDCPrinterPtr itfPrinter = pUDC->Printers["Universal Document Converter"]; UDC::IProfilePtr itfProfile = itfPrinter->Profile; // Use Universal Document Converter API to change settings of converterd document // Load profile located in folder "%APPDATA%\UDC Profiles". // Value of %APPDATA% variable should be received using Windows API's // SHGetSpecialFolderPath function. Or you can move default profiles into a folder // you prefer. itfProfile->Load("Drawing to TIFF.xml"); itfProfile->OutputLocation->Mode = UDC::LM_PREDEFINED; itfProfile->OutputLocation->FolderPath = L"C:\\Out"; itfProfile->PostProcessing->Mode = UDC::PP_OPEN_FOLDER; // Run Microsoft Visio as COM-server VISIO::IVApplicationPtr objVisioApp( L"Visio.Application" ); VISIO::IVDocumentPtr itfDrawing; objVisioApp->Visible = false; // Open drawing from file itfDrawing = objVisioApp->Documents->OpenEx( (LPCTSTR)sFilePath, visOpenRW | visOpenDontList | visOpenMacrosDisabled | visOpenHidden ); // Change Drawing preferences for scaling it to page itfDrawing->PrintCenteredH = true; itfDrawing->PrintCenteredV = true; itfDrawing->PrintFitOnPages = true; // Print drawing itfDrawing->Printer = "Universal Document Converter"; itfDrawing->Print(); // Close drawing itfDrawing->Saved = true; itfDrawing->Close(); // Close Microsoft Visio objVisioApp->Quit(); }
Visual C#
C# Visio to TIFF conversion code example:
/////////////////////////////////////////////////////////////////////////////////// // This example was designed to be used in Microsoft Visual C# from // Microsoft Visual Studio version 2003 or higher. // // 1. Microsoft Visio version 2002 or higher should be installed and activated // on your PC // // 2. Universal Document Converter 5.2 or higher should be installed as well // // 3. Add references to Microsoft Visio XX.0 Object Library and // Universal Document Converter Type Library using // the Project | Add Reference menu > COM tab. // XX is the Microsoft Office version installed on your computer. /////////////////////////////////////////////////////////////////////////////////// using System; using System.IO; using UDC; using Visio = Microsoft.Office.Interop.Visio; namespace VisioToTIFF { class Program { static void PrintVisioToTIFF(string VisioFilePath) { //Create a UDC object and get its interfaces IUDC objUDC = new APIWrapper(); IUDCPrinter Printer = objUDC.get_Printers("Universal Document Converter"); IProfile Profile = Printer.Profile; //Use Universal Document Converter API to change settings of //converterd document //Load profile located in folder "%APPDATA%\UDC Profiles". //Value of %APPDATA% variable should be received using //Environment.GetFolderPath method. Or you can move default profiles into //a folder you prefer. string AppDataPath = Environment.GetFolderPath( Environment.SpecialFolder.ApplicationData); string ProfilePath = Path.Combine(AppDataPath, @"UDC Profiles\Drawing to TIFF.xml"); Profile.Load(ProfilePath); Profile.OutputLocation.Mode = LocationModeID.LM_PREDEFINED; Profile.OutputLocation.FolderPath = @"c:\UDC Output Files"; Profile.PostProcessing.Mode = PostProcessingModeID.PP_OPEN_FOLDER; //Run Microsoft Visio as COM-server Visio.Application VisioApp = new Visio.ApplicationClass(); VisioApp.Visible = true; //Open document from file short Flags = (short)(Visio.VisOpenSaveArgs.visOpenRW | Visio.VisOpenSaveArgs.visOpenDontList | Visio.VisOpenSaveArgs.visOpenMacrosDisabled | Visio.VisOpenSaveArgs.visOpenHidden); Visio.Document Document = VisioApp.Documents.OpenEx(VisioFilePath, Flags); //Change document preferences for scaling it to page Document.PrintCenteredH = true; Document.PrintCenteredV = true; Document.PrintFitOnPages = true; //Print document Document.Printer = "Universal Document Converter"; Document.Print(); //Close document Document.Saved = true; Document.Close(); //Close Visio VisioApp.Quit(); } static void Main(string[] args) { string TestFilePath = Path.Combine(AppDomain.CurrentDomain.BaseDirectory, "TestFile.vsd"); PrintVisioToTIFF(TestFilePath); } } }
Delphi
Delphi Visio to TIFF conversion code example:
////////////////////////////////////////////////////////////////////////////////// // This example was designed to be used in Delphi version 7 or higher. // // 1. Microsoft Visio version 2002 or higher should be installed and activated // on your PC // // 2. Universal Document Converter version 5.2 or higher should be installed as well // // 3. Add Universal Document Converter Type Library and // Microsoft Visio XX.0 Object Library type libraries to the project. // XX is the Microsoft Office version installed on your computer. // // Delphi 7: // Use the Project | Import Type Library menu. // // Delphi version 2006 or higher: // Use the Component | Import Component menu. // // Clear the Generate Component Wrapper checkbox and click the Create Unit button // (Delphi 7) or select the Create Unit option (Delphi 2006 or latter). // // 4. Notice that the number of Microsoft Visio method parameters may depend // on the Visio version. ////////////////////////////////////////////////////////////////////////////////// program VisioToTIFF; {$APPTYPE CONSOLE} uses SysUtils, Variants, Windows, Dialogs, ActiveX, UDC_TLB, Visio_TLB; procedure PrintVisioToTIFF(VisioFilePath: string); var objUDC: IUDC; Printer: IUDCPrinter; Profile: IProfile; VisioApp: IVApplication; Document: IVDocument; Flags: SmallInt; begin //Create a UDC object and get its interfaces objUDC := CoAPIWrapper.Create; Printer := objUDC.get_Printers('Universal Document Converter'); Profile := Printer.Profile; //Use Universal Document Converter API to change settings of converterd document //Load profile located in folder "%APPDATA%\UDC Profiles". //Value of %APPDATA% variable should be received using Windows API's // SHGetSpecialFolderPath or JCL's JclSysInfo.GetAppdataFolder function. //Or you can move default profiles into a folder you prefer. Profile.Load('Drawing to TIFF.xml'); Profile.OutputLocation.Mode := LM_PREDEFINED; Profile.OutputLocation.FolderPath := 'c:\UDC Output Files'; Profile.PostProcessing.Mode := PP_OPEN_FOLDER; //Run Microsoft Visio as COM-server VisioApp := CoVisioApplication.Create; VisioApp.Visible := True; //Open document from file Flags := visOpenRW or visOpenDontList or visOpenMacrosDisabled or visOpenHidden; Document := VisioApp.Documents.OpenEx(VisioFilePath, Flags); //Change document preferences for scaling it to page Document.PrintCenteredH := True; Document.PrintCenteredV := True; Document.PrintFitOnPages := True; //Print document Document.Printer := 'Universal Document Converter'; Document.Print(); //Close document Document.Saved := True; Document.Close(); //Close Visio VisioApp.Quit(); end; var TestFilePath: string; begin TestFilePath := ExtractFilePath(ParamStr(0)) + 'TestFile.vsd'; try CoInitialize(nil); try PrintVisioToTIFF(TestFilePath); finally CoUninitialize; end; except on E: Exception do MessageDlg(E.ClassName + ' : ' + E.Message, mtError, [mbOK], 0); end; end.
PHP
PHP Visio to TIFF converson code example:
'---------------------------------------------------------------------- ' 1) Microsoft Visio 2000 or above should be installed and activated on your PC. ' ' 2) Universal Document Converter 5.2 or above should also be installed. ' ' 3) Apache WEB server and PHP 4.0 or above should be installed and adjusted. '---------------------------------------------------------------------- <?PHP //Create Universal Document Converter object $objUDC = new COM("UDC.APIWrapper"); //Set up Universal Document Converter $itfPrinter = $objUDC->Printers("Universal Document Converter"); $itfProfile = $itfPrinter->Profile; $itfProfile->PageSetup->ResolutionX = 300; $itfProfile->PageSetup->ResolutionY = 300; $itfProfile->PageSetup->Orientation = 0; $itfProfile->PageSetup->Units = 1; $itfProfile->PageSetup->Width = 220; $itfProfile->PageSetup->Height = 180; $itfProfile->FileFormat->ActualFormat = 3; $itfProfile->FileFormat->TIFF->ColorSpace = 24; $itfProfile->FileFormat->TIFF->Compression = 3; $itfProfile->FileFormat->TIFF->Multipage = 2; $itfProfile->FileFormat->TIFF->Smoothing = 1; $itfProfile->OutputLocation->Mode = 1; $itfProfile->OutputLocation->FolderPath = '&[Documents]\UDC Output Files\\'; $itfProfile->OutputLocation->FileName = '&[DocName(0)].&[ImageType]'; $itfProfile->OutputLocation->OverwriteExistingFile = 1; $itfProfile->Adjustments->Crop->Mode = 0; $itfProfile->PostProcessing->Mode = 0; $itProfile->ShowProgressWnd = 1; //Create MS Visio object and open the file $file = 'my_scheme.vsd'; $VisioApp = new COM("Visio.Application"); $Drawing = $VisioApp->Documents->Open($file); //Change the preferences of drawing $Drawing->PrintCenteredH = True; $Drawing->PrintCenteredV = True; $Drawing->PrintFitOnPages = True; //Printing $Drawing->Printer = "Universal Document Converter"; $Drawing->PrintOut(0); //Close the document $Drawing->Saved = True; //Close Visio $VisioApp->Quit; echo "READY!"; ?>
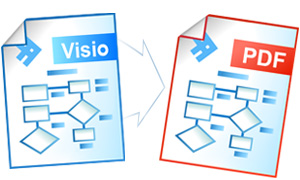