Automatic Conversion from AutoCAD to PDF
Universal Document Converter can be used for converting your documents into PDF, JPEG, TIFF, or PNG format. Software developers can utilize our resources and control the existing settings of the Universal Document Converter using COM-interface and using Autodesk AutoCAD as COM-server for printing.
Universal Document Converter is virtual printer software that saves any document you print as PDF or as an image file such as JPEG, TIFF or PNG. As a software developer, you can manage Universal Document Converter settings using COM-interface and use Autodesk AutoCAD as COM-server for printing drawings to PDF.
AutoCAD drawing conversion source code examples:
Visual Basic.NET
VB.NET AutoCAD to PDF converter code example:
'---------------------------------------------------------------------- ' 1) Autodesk AutoCAD 2000 or above should be installed and activated on your PC ' ' 2) Universal Document Converter 5.2 or above should be installed, too ' ' 3) Open your project in Microsoft Visual Basic.NET ' ' 4) In Visual Basic main menu press Project->Add Reference... ' ' 5) In Add Reference window go to COM tab and double click into ' Universal Document Converter Type Library '---------------------------------------------------------------------- Private Sub PrintACADToPDF(ByVal strFilePath As String) ' Define constants Const acExtents = 1 Const acScaleToFit = 0 Dim nBACKGROUNDPLOT, nFILEDIA, nCMDDIA As Long Dim objUDC As UDC.IUDC Dim itfPrinter As UDC.IUDCPrinter Dim itfProfile As UDC.IProfile Dim objACADApp As Object Dim itfDrawing As Object Dim itfLayout As Object Dim itfActiveSpace As Object Dim AppDataPath As String Dim ProfilePath As String objUDC = New UDC.APIWrapper itfPrinter = objUDC.Printers("Universal Document Converter") itfProfile = itfPrinter.Profile ' Use Universal Document Converter API to change settings of converterd document ' Load profile located in folder "%APPDATA%\UDC Profiles". ' Value of %APPDATA% variable should be received using Environment.GetFolderPath ' method. Or you can move default profiles into a folder you prefer. AppDataPath = Environment.GetFolderPath(Environment.SpecialFolder.ApplicationData) ProfilePath = Path.Combine(AppDataPath, "UDC Profiles\Drawing to PDF.xml") itfProfile.Load(ProfilePath) itfProfile.OutputLocation.Mode = UDC.LocationModeID.LM_PREDEFINED itfProfile.OutputLocation.FolderPath = "C:\Out" itfProfile.PostProcessing.Mode = UDC.PostProcessingModeID.PP_OPEN_FOLDER ' Run AutoCAD as COM-server On Error Resume Next objACADApp = CreateObject("AutoCAD.Application") ' Open drawing from file itfDrawing = objACADApp.Documents.Open(strFilePath, False) ' Change AutoCAD preferences for scaling the drawing to page If itfDrawing.ActiveSpace = 0 Then itfActiveSpace = itfDrawing.PaperSpace itfLayout = itfActiveSpace.Layout Else itfActiveSpace = itfDrawing.ModelSpace itfLayout = itfActiveSpace.Layout End If itfLayout.PlotType = acExtents itfLayout.UseStandardScale = True itfLayout.StandardScale = acScaleToFit itfLayout.CenterPlot = True nBACKGROUNDPLOT = itfDrawing.GetVariable("BACKGROUNDPLOT") nFILEDIA = itfDrawing.GetVariable("FILEDIA") nCMDDIA = itfDrawing.GetVariable("CMDDIA") Call itfDrawing.SetVariable("BACKGROUNDPLOT", 0) Call itfDrawing.SetVariable("FILEDIA", 0) Call itfDrawing.SetVariable("CMDDIA", 0) itfDrawing.Plot.QuietErrorMode = True ' Plot the drawing Call itfDrawing.Plot.PlotToDevice("Universal Document Converter") ' Restore AutoCAD default preferences Call itfDrawing.SetVariable("BACKGROUNDPLOT", nBACKGROUNDPLOT) Call itfDrawing.SetVariable("FILEDIA", nFILEDIA) Call itfDrawing.SetVariable("CMDDIA", nCMDDIA) ' Close drawing Call itfDrawing.Close(False) itfDrawing = Nothing ' Close Autodesk AutoCAD Call objACADApp.Quit() objACADApp = Nothing End Sub
Visual Basic 6
VB AutoCAD to PDF converter code example:
'---------------------------------------------------------------------- ' 1) Autodesk AutoCAD 2000 or above should be installed and activated on your PC ' ' 2) Universal Document Converter 5.2 or above should be installed, too ' ' 3) Open your project in Microsoft Visual Basic 6.0 ' ' 4) In Visual Basic main menu press Project->References ' ' 5) In the list of references check Universal Document Converter Type Library '---------------------------------------------------------------------- Private Sub PrintACADToPDF(strFilePath As String) ' Define constants Const acExtents = 1 Const acScaleToFit = 0 Dim nBACKGROUNDPLOT, nFILEDIA, nCMDDIA As Long Dim objUDC As IUDC Dim itfPrinter As IUDCPrinter Dim itfProfile As IProfile Dim objACADApp As Object Dim itfDrawing As Object Dim itfLayout As Object Dim itfActiveSpace As Object Set objUDC = New UDC.APIWrapper Set itfPrinter = objUDC.Printers("Universal Document Converter") Set itfProfile = itfPrinter.Profile ' Use Universal Document Converter API to change settings of converterd document ' Load profile located in folder "%APPDATA%\UDC Profiles". ' Value of %APPDATA% variable should be received using Windows API's ' SHGetSpecialFolderPath function. Or you can move default profiles into a ' folder you prefer. itfProfile.Load("Drawing to PDF.xml") itfProfile.OutputLocation.Mode = LM_PREDEFINED itfProfile.OutputLocation.FolderPath = "C:\Out" itfProfile.PostProcessing.Mode = PP_OPEN_FOLDER ' Run AutoCAD as COM-server On Error Resume Next Set objACADApp = CreateObject("AutoCAD.Application") ' Open drawing from file Err = 0 ' Clear GetLastError() value Set itfDrawing = objACADApp.Documents.Open(strFilePath, False) If Err = 0 Then ' Change AutoCAD preferences for scaling the drawing to page If itfDrawing.ActiveSpace = 0 Then Set itfActiveSpace = itfDrawing.PaperSpace Set itfLayout = itfActiveSpace.Layout Else Set itfActiveSpace = itfDrawing.ModelSpace Set itfLayout = itfActiveSpace.Layout End If itfLayout.PlotType = acExtents itfLayout.UseStandardScale = True itfLayout.StandardScale = acScaleToFit itfLayout.CenterPlot = True nBACKGROUNDPLOT = itfDrawing.GetVariable("BACKGROUNDPLOT") nFILEDIA = itfDrawing.GetVariable("FILEDIA") nCMDDIA = itfDrawing.GetVariable("CMDDIA") Call itfDrawing.SetVariable("BACKGROUNDPLOT", 0) Call itfDrawing.SetVariable("FILEDIA", 0) Call itfDrawing.SetVariable("CMDDIA", 0) itfDrawing.Plot.QuietErrorMode = True ' Plot the drawing Call itfDrawing.Plot.PlotToDevice("Universal Document Converter") ' Restore AutoCAD default preferences Call itfDrawing.SetVariable("BACKGROUNDPLOT", nBACKGROUNDPLOT) Call itfDrawing.SetVariable("FILEDIA", nFILEDIA) Call itfDrawing.SetVariable("CMDDIA", nCMDDIA) ' Close drawing Call itfDrawing.Close(False) Set itfDrawing = Nothing End If ' Close Autodesk AutoCAD Call objACADApp.Quit Set objACADApp = Nothing End Sub
Visual C++
C++ AutoCAD to PDF converter code example:
////////////////////////////////////////////////////////////////// // This example was designed for using in Microsoft Visual C++ from // Microsoft Visual Studio 2003 or above. // // 1. Autodesk AutoCAD 2000 or above should be installed and activated on your PC. // Autodesk AutoCAD LT does not have COM interface and cannot be used as COM-server! // // 2. Universal Document Converter 5.2 or above should be installed, too. // // 3. You must initialize the COM before you call any COM method. // Please insert ::CoInitialize(0); in your application initialization // and ::CoUninitialize(); before closing it. // // 4. Import AutoCAD libraries for 32-bit version of Windows. // For 64-bit version please change C:\\Program Files\\ to // C:\\Program Files (x86)\\ in all pathes. #pragma message("Import AutoCAD API") // AutoCAD 2000 -> "C:\\Program Files\\ACAD2000\\acad.tlb" // AutoCAD 2002 -> "C:\\Program Files\\AutoCAD 2002\\acad.tlb" // AutoCAD 2004 -> "C:\\Program Files\\Common Files\\Autodesk Shared\\acax16enu.tlb" // AutoCAD 2005 -> "C:\\Program Files\\Common Files\\Autodesk Shared\\acax16enu.tlb" // AutoCAD 2006 -> "C:\\Program Files\\Common Files\\Autodesk Shared\\acax16enu.tlb" // AutoCAD 2007 -> "C:\\Program Files\\Common Files\\Autodesk Shared\\acax17enu.tlb" // AutoCAD 2008 -> "C:\\Program Files\\Common Files\\Autodesk Shared\\acax17enu.tlb" // AutoCAD 2009 -> "C:\\Program Files\\Common Files\\Autodesk Shared\\acax17enu.tlb" #import "C:\\Program Files\\Common Files\\Autodesk Shared\\acax17enu.tlb"\ rename_namespace("ACAD"), auto_rename // 5. Import Universal Document Converter software API: #import "progid:udc.apiwrapper" rename_namespace("UDC") ////////////////////////////////////////////////////////////////// void PrintAdobePDFToJPEG( CString sFilePath ) { LONG nBACKGROUNDPLOT, nFILEDIA, nCMDDIA; float fAppVer = 0.f; UDC::IUDCPtr pUDC(__uuidof(UDC::APIWrapper)); UDC::IUDCPrinterPtr itfPrinter = pUDC->Printers["Universal Document Converter"]; UDC::IProfilePtr itfProfile = itfPrinter->Profile; // Use Universal Document Converter API to change settings of converterd drawing // Load profile located in folder "%APPDATA%\UDC Profiles". // Value of %APPDATA% variable should be received using Windows API's // SHGetSpecialFolderPath function. Or you can move default profiles into // a folder you prefer. itfProfile->Load("Drawing to PDF.xml"); itfProfile->OutputLocation->Mode = UDC::LM_PREDEFINED; itfProfile->OutputLocation->FolderPath = L"C:\\Out"; itfProfile->PostProcessing->Mode = UDC::PP_OPEN_FOLDER; // Run AutoCAD as COM-server ACAD::IAcadApplicationPtr objACADApp(L"AutoCAD.Application"); ACAD::IAcadDocumentPtr itfDrawing; ACAD::IAcadLayoutPtr itfLayout; ACAD::IAcadPaperSpacePtr itfActiveSpace; sscanf_s( objACADApp->Version, "%f", &fAppVer ); // Open drawing from file itfDrawing = objACADApp->Documents->Open( (LPCTSTR)sFilePath, false ); // Change AutoCAD preferences for scaling the drawing to page if( itfDrawing->ActiveSpace == 0 ) { itfActiveSpace = itfDrawing->PaperSpace; itfLayout = itfActiveSpace->Layout; } else { itfActiveSpace = itfDrawing->ModelSpace; itfLayout = itfActiveSpace->Layout; } itfLayout->PlotType = ACAD::acExtents; itfLayout->UseStandardScale = true; itfLayout->StandardScale = ACAD::acScaleToFit; itfLayout->CenterPlot = true; if( fAppVer >= 16.1f ) { nBACKGROUNDPLOT = itfDrawing->GetVariable("BACKGROUNDPLOT"); nFILEDIA = itfDrawing->GetVariable("FILEDIA"); nCMDDIA = itfDrawing->GetVariable("CMDDIA"); itfDrawing->SetVariable("BACKGROUNDPLOT", 0L); itfDrawing->SetVariable("FILEDIA", 0L); itfDrawing->SetVariable("CMDDIA", 0L); } itfDrawing->Plot->QuietErrorMode = true; // Plot the drawing itfDrawing->Plot->PlotToDevice("Universal Document Converter"); if( fAppVer >= 16.1f ) { // Restore AutoCAD default preferences itfDrawing->SetVariable("BACKGROUNDPLOT", nBACKGROUNDPLOT); itfDrawing->SetVariable("FILEDIA", nFILEDIA); itfDrawing->SetVariable("CMDDIA", nCMDDIA); } // Close drawing itfDrawing->Close(false); // Close Autodesk AutoCAD objACADApp->Quit(); }
Visual C#
C# AutoCAD to PDF converter code example:
///////////////////////////////////////////////////////////////////////////////// // This example was designed for using in Microsoft Visual C# from // Microsoft Visual Studio 2003 or above. // // 1. Autodesk AutoCAD 2000 or above should be installed and activated on your PC. // Autodesk AutoCAD LT does not have COM interface and cannot be used as COM-server! // // 2. Universal Document Converter 5.2 or above should be installed, too. // // 3. Add references to AutoCAD 2006 Type Library, // AutoCAD/ObjectDBX Common 16.0 Type Library and // Universal Document Converter Type Library using the // Project | Add Reference menu > COM tab. // // The version numbers in the type library names may be different depending on // AutoCAD's version installed on your computer. ///////////////////////////////////////////////////////////////////////////////// using System; using System.IO; using System.Globalization; using UDC; using AutoCAD = Autodesk.AutoCAD.Interop; namespace AutoCADtoPDF { class Program { static void PrintAutoCADtoPDF(string AutoCADFilePath) { //Create a UDC object and get its interfaces IUDC objUDC = new APIWrapper(); IUDCPrinter Printer = objUDC.get_Printers("Universal Document Converter"); IProfile Profile = Printer.Profile; //Use Universal Document Converter API to change settings of //converterd drawing //Load profile located in folder "%APPDATA%\UDC Profiles". //Value of %APPDATA% variable should be received using //Environment.GetFolderPath method. //Or you can move default profiles into a folder you prefer. string AppDataPath = Environment.GetFolderPath( Environment.SpecialFolder.ApplicationData); string ProfilePath = Path.Combine(AppDataPath, @"UDC Profiles\Drawing to PDF.xml"); Profile.Load(ProfilePath); Profile.OutputLocation.Mode = LocationModeID.LM_PREDEFINED; Profile.OutputLocation.FolderPath = @"c:\UDC Output Files"; Profile.PostProcessing.Mode = PostProcessingModeID.PP_OPEN_FOLDER; AutoCAD.AcadApplication App = new AutoCAD.AcadApplicationClass(); double Version = double.Parse(App.Version.Substring(0, 4), new CultureInfo("en-US")); //Open drawing from file Object ReadOnly = false; Object Password = Type.Missing; AutoCAD.AcadDocument Doc = App.Documents.Open(AutoCADFilePath, ReadOnly, Password); //AutoCAD.Common.AcadPaperSpace ActiveSpace; AutoCAD.Common.AcadLayout Layout; //Change AutoCAD preferences for scaling the drawing to page if(Doc.ActiveSpace == 0) Layout = Doc.PaperSpace.Layout; else Layout = Doc.ModelSpace.Layout; Layout.PlotType = AutoCAD.Common.AcPlotType.acExtents; Layout.UseStandardScale = true; Layout.StandardScale = AutoCAD.Common.AcPlotScale.acScaleToFit; Layout.CenterPlot = true; Object nBACKGROUNDPLOT = 0, nFILEDIA = 0, nCMDDIA = 0; if(Version >= 16.1f) { nBACKGROUNDPLOT = Doc.GetVariable("BACKGROUNDPLOT"); nFILEDIA = Doc.GetVariable("FILEDIA"); nCMDDIA = Doc.GetVariable("CMDDIA"); Object xNull = 0; Doc.SetVariable("BACKGROUNDPLOT", xNull); Doc.SetVariable("FILEDIA", xNull); Doc.SetVariable("CMDDIA", xNull); } Doc.Plot.QuietErrorMode = true; //Plot the drawing Doc.Plot.PlotToDevice("Universal Document Converter"); if(Version >= 16.1f ) { //Restore AutoCAD default preferences Doc.SetVariable("BACKGROUNDPLOT", nBACKGROUNDPLOT); Doc.SetVariable("FILEDIA", nFILEDIA); Doc.SetVariable("CMDDIA", nCMDDIA); } //Close drawing Object SaveChanges = false; Doc.Close(SaveChanges, Type.Missing); //Close Autodesk AutoCAD App.Quit(); } static void Main(string[] args) { string TestFilePath = Path.Combine(AppDomain.CurrentDomain.BaseDirectory, "TestFile.dwg"); PrintAutoCADtoPDF(TestFilePath); } } }
Delphi
Delphi AutoCAD to PDF converter code example:
///////////////////////////////////////////////////////////////////////////////////// // This example was designed for using in Delphi 7 or higher. // // 1. Autodesk AutoCAD 2000 or above should be installed and activated on your PC. // Autodesk AutoCAD LT does not have COM interface and cannot be used as COM-server! // // 2. Universal Document Converter 5.2 or above should be installed, too. // // 3. Add Universal Document Converter Type Library and AutoCAD XXXX Type Library // type libraries to the project. XXXX is the AutoCAD version installed on your PC // // Delphi 7: // Use the Project | Import Type Library menu. // // Delphi 2006 or latter: // Use the Component | Import Component menu. // // Clear the Generate Component Wrapper checkbox and click the Create Unit // button (Delphi 7) or select the Create Unit option (Delphi 2006 or latter). // ///////////////////////////////////////////////////////////////////////////////////// program AutoCADtoPDF; {$APPTYPE CONSOLE} uses SysUtils, Variants, Windows, Dialogs, ActiveX, UDC_TLB, AXDBLib_TLB, AutoCAD_TLB; procedure PrintAutoCADtoPDF(AutoCADFilePath: string); var objUDC: IUDC; Printer: IUDCPrinter; Profile: IProfile; App: AcadApplication; Doc: AcadDocument; Layout: AcadLayout; Version: Double; FormatSettings: TFormatSettings; ReadOnly, Password: OleVariant; nBACKGROUNDPLOT, nFILEDIA, nCMDDIA: OleVariant; xNull: OleVariant; SaveChanges: OleVariant; begin //Create a UDC object and get its interfaces objUDC := CoAPIWrapper.Create; Printer := objUDC.get_Printers('Universal Document Converter'); Profile := Printer.Profile; //Use Universal Document Converter API to change settings of converterd drawing //Load profile located in folder "%APPDATA%\UDC Profiles". //Value of %APPDATA% variable should be received using Windows API's SHGetSpecialFolderPath or JCL's JclSysInfo.GetAppdataFolder function. //Or you can move default profiles into a folder you prefer. Profile.Load('Drawing to PDF.xml'); Profile.OutputLocation.Mode := LM_PREDEFINED; Profile.OutputLocation.FolderPath := 'c:\UDC Output Files'; Profile.PostProcessing.Mode := PP_OPEN_FOLDER; App := CoAcadApplication.Create; GetLocaleFormatSettings($0409 {en_US}, FormatSettings); Version := StrToFloat(Copy(App.Version, 1, 4), FormatSettings); //Open drawing from file ReadOnly := False; Password := Variants.EmptyParam; Doc := App.Documents.Open(AutoCADFilePath, ReadOnly, Password); //Change AutoCAD preferences for scaling the drawing to page if Doc.ActiveSpace = acPaperSpace then Layout := Doc.PaperSpace.Layout else //Doc.ActiveSpace = acModelSpace Layout := Doc.ModelSpace.Layout; Layout.PlotType := acExtents; Layout.UseStandardScale := True; Layout.StandardScale := acScaleToFit; Layout.CenterPlot := True; nBACKGROUNDPLOT := 0; nFILEDIA := 0; nCMDDIA := 0; if Version >= 16.1 then begin nBACKGROUNDPLOT := Doc.GetVariable('BACKGROUNDPLOT'); nFILEDIA := Doc.GetVariable('FILEDIA'); nCMDDIA := Doc.GetVariable('CMDDIA'); xNull := 0; Doc.SetVariable('BACKGROUNDPLOT', xNull); Doc.SetVariable('FILEDIA', xNull); Doc.SetVariable('CMDDIA', xNull); end; Doc.Plot.QuietErrorMode := True; //Plot the drawing Doc.Plot.PlotToDevice('Universal Document Converter'); if Version >= 16.1 then begin //Restore AutoCAD default preferences Doc.SetVariable('BACKGROUNDPLOT', nBACKGROUNDPLOT); Doc.SetVariable('FILEDIA', nFILEDIA); Doc.SetVariable('CMDDIA', nCMDDIA); end; //Close drawing SaveChanges := False; Doc.Close(SaveChanges, Variants.EmptyParam); //Close Autodesk AutoCAD App.Quit(); end; var TestFilePath: string; begin TestFilePath := ExtractFilePath(ParamStr(0)) + 'TestFile.dwg'; try CoInitialize(nil); try PrintAutoCADtoPDF(TestFilePath); finally CoUninitialize; end; except on E: Exception do MessageDlg(E.ClassName + ' : ' + E.Message, mtError, [mbOK], 0); end; end.
PHP
PHP AutoCAD to PDF converter code example:
'---------------------------------------------------------------------- ' 1) Autodesk AutoCAD 2000 or above should be installed and activated on your PC. ' ' 2) Universal Document Converter 5.2 or above should also be installed. ' ' 3) Apache WEB server and PHP 4.0 or above should be installed and adjusted. '---------------------------------------------------------------------- <?PHP //Create Universal Document Converter object $objUDC = new COM("UDC.APIWrapper"); //Set up Universal Document Converter $itfPrinter = $objUDC->Printers("Universal Document Converter"); $itfProfile = $itfPrinter->Profile; $itfProfile->PageSetup->ResolutionX = 300; $itfProfile->PageSetup->ResolutionY = 300; $itfProfile->PageSetup->Orientation = 0; $itfProfile->FileFormat->ActualFormat = 7; $itfProfile->FileFormat->PDF->ColorSpace = 24; $itfProfile->FileFormat->PDF->Compression = 4; $itfProfile->FileFormat->PDF->Multipage = 2; $itfProfile->OutputLocation->Mode = 1; $itfProfile->OutputLocation->FolderPath = '&[Documents]\UDC Output Files\\'; $itfProfile->OutputLocation->FileName = '&[DocName(0)].&[ImageType]'; $itfProfile->OutputLocation->OverwriteExistingFile = 1; $itfProfile->Adjustments->Crop->Mode = 0; $itfProfile->PostProcessing->Mode = 0; $itProfile->ShowProgressWnd = 1; //Create AutoCAD object and open .DWG file $file = 'my_chart.dwg'; $ACADApp = new COM("AutoCAD.Application"); $Drawing = $ACADApp->Documents->Open($file,false); //Change the preferences of AutoCAD for scaling the drawing to page If ($Drawing->ActiveSpace = 0){ $Layout = $Drawing->PaperSpace->Layout; } Else { $Layout = $Drawing->ModelSpace->Layout; } $Layout->PlotType = 1; $Layout->UseStandardScale = True; $Layout->StandardScale = 0; $Layout->CenterPlot = True; $Drawing->SetVariable("BACKGROUNDPLOT", 0); $Drawing->SetVariable("FILEDIA", 0); $Drawing->SetVariable("CMDDIA", 0); // Plot the drawing $Drawing->Plot->QuietErrorMode = True; $Drawing->Plot->PlotToDevice("Universal Document Converter"); //Pause for processing sleep(5); // Close drawing $Drawing->Close(False); //Close Autodesk AutoCAD $ACADApp->Quit(); echo "READY!"; ?>
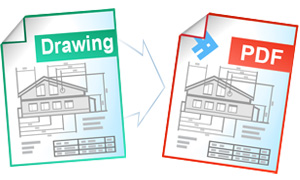